Customize the WordPress Admin Toolbar: Why and How To Do It
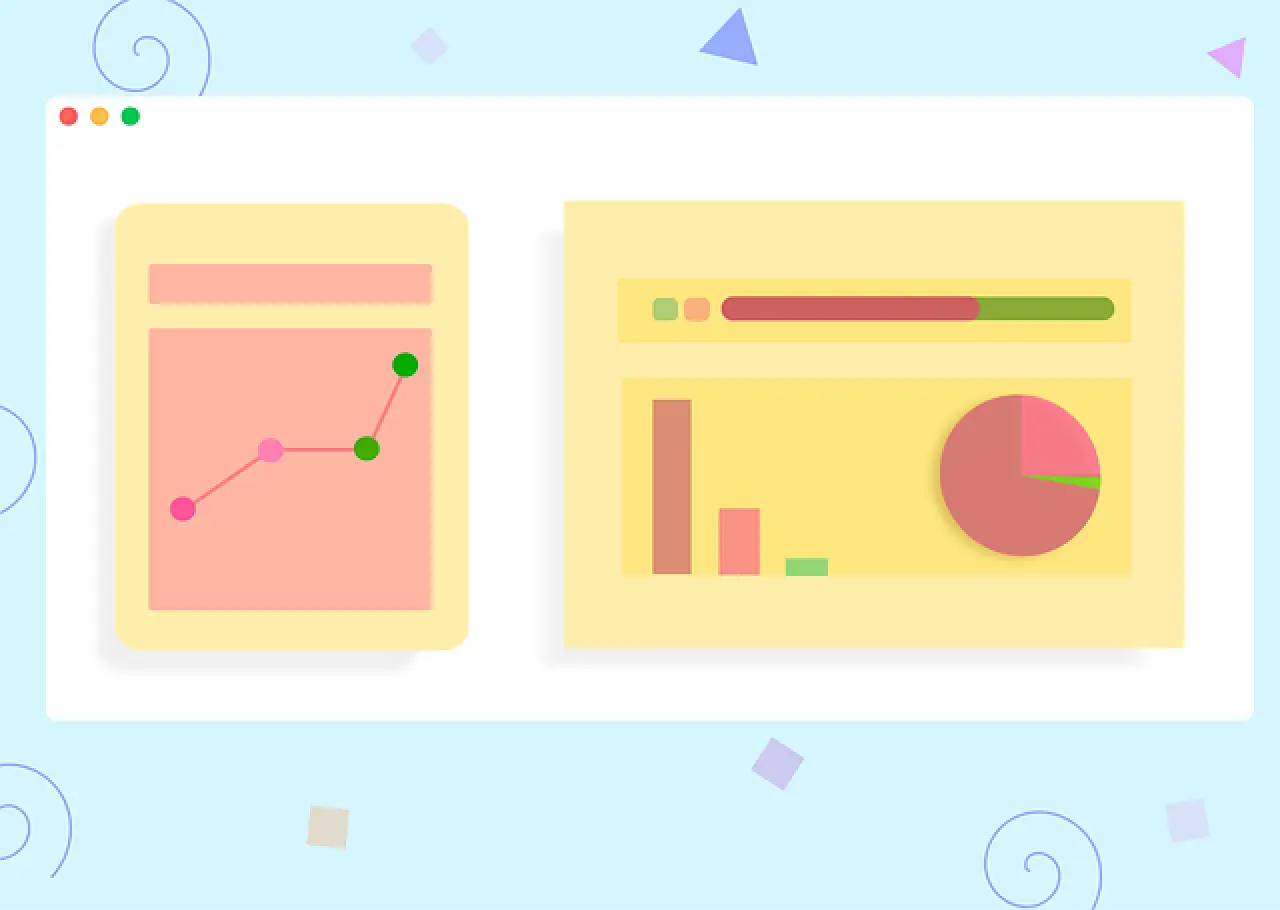
The WordPress admin toolbar, sometimes known as the admin bar, serves as a convenient shortcut for your WordPress website, providing you with quick access to various settings, creating content, and more. However, not all users or developers find the default options sufficient or relevant to their workflow. This is why learning how to customize the WordPress admin bar can be incredibly beneficial.
In this comprehensive guide, we will explore why you might want to customize the admin bar, the methods to do it, including the use of plugins and code snippets, and how to troubleshoot common issues such as the missing admin bar problem. Let's delve in.
TABLE OF CONTENTS
- Why Customize the WordPress Admin Bar?
- How to Customize the WordPress Admin Toolbar
- Customizing the Appearance of the Toolbar
- Toolbar Permissions: Managing Who Can See What
- Using the Toolbar in Development
- Disabling the Toolbar
- How to Hide Admin Bar for Mobile Users
- How to Add Custom Logo to the Admin Bar
- Troubleshooting Common Issues
- Managing Admin Bar Visibility
- WordPress Database Errors
- Conclusion
Why Customize the WordPress Admin Bar?
The WordPress admin toolbar sits at the top of the WordPress dashboard and the front end of your site, offering quick access to various admin tasks. However, there might be menus and options you rarely use. Sometimes, you might wish the admin bar shows different settings or shortcuts that align better with your workflow. This is where customization comes in.
Why should we customize bar?
For instance, if you're a developer working on a client's WordPress website, you might want to add a menu item that directs you to a particular WordPress theme file you're constantly tweaking. Conversely, you might want to hide the WP admin bar for all other users, except administrators to streamline the interface.
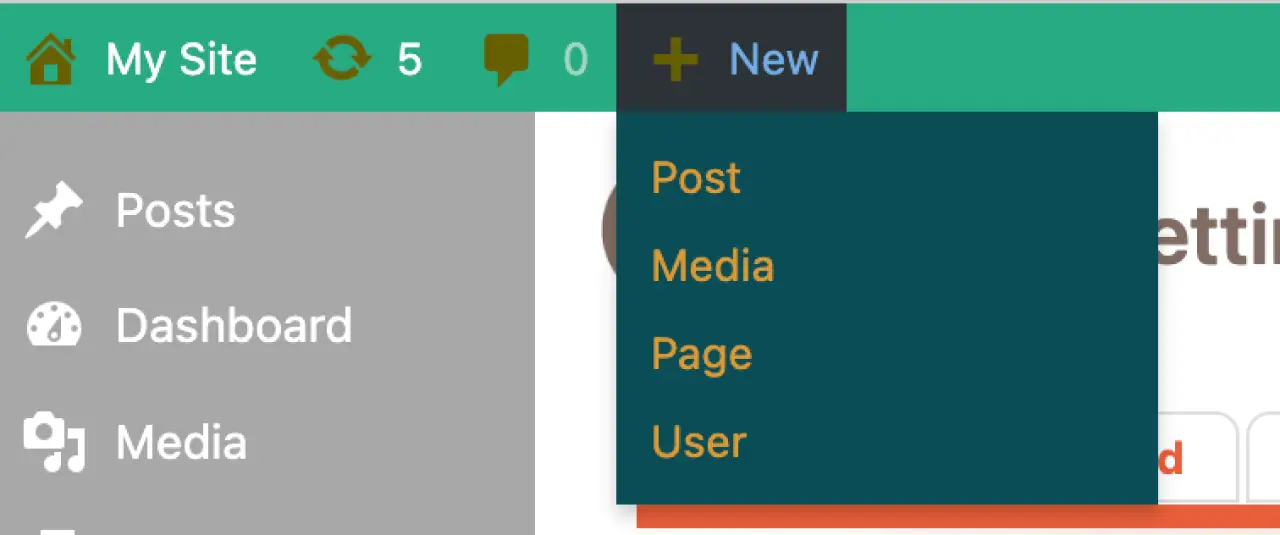
In essence, learning how to customize the WordPress admin bar allows you to tailor your WordPress admin area to your specific needs, potentially making you more efficient at managing your WordPress site.
How to Customize the WordPress Admin Toolbar
There are several methods to customize the WordPress admin toolbar, from plugins to writing your own code. Below, we'll explore both methods in detail.
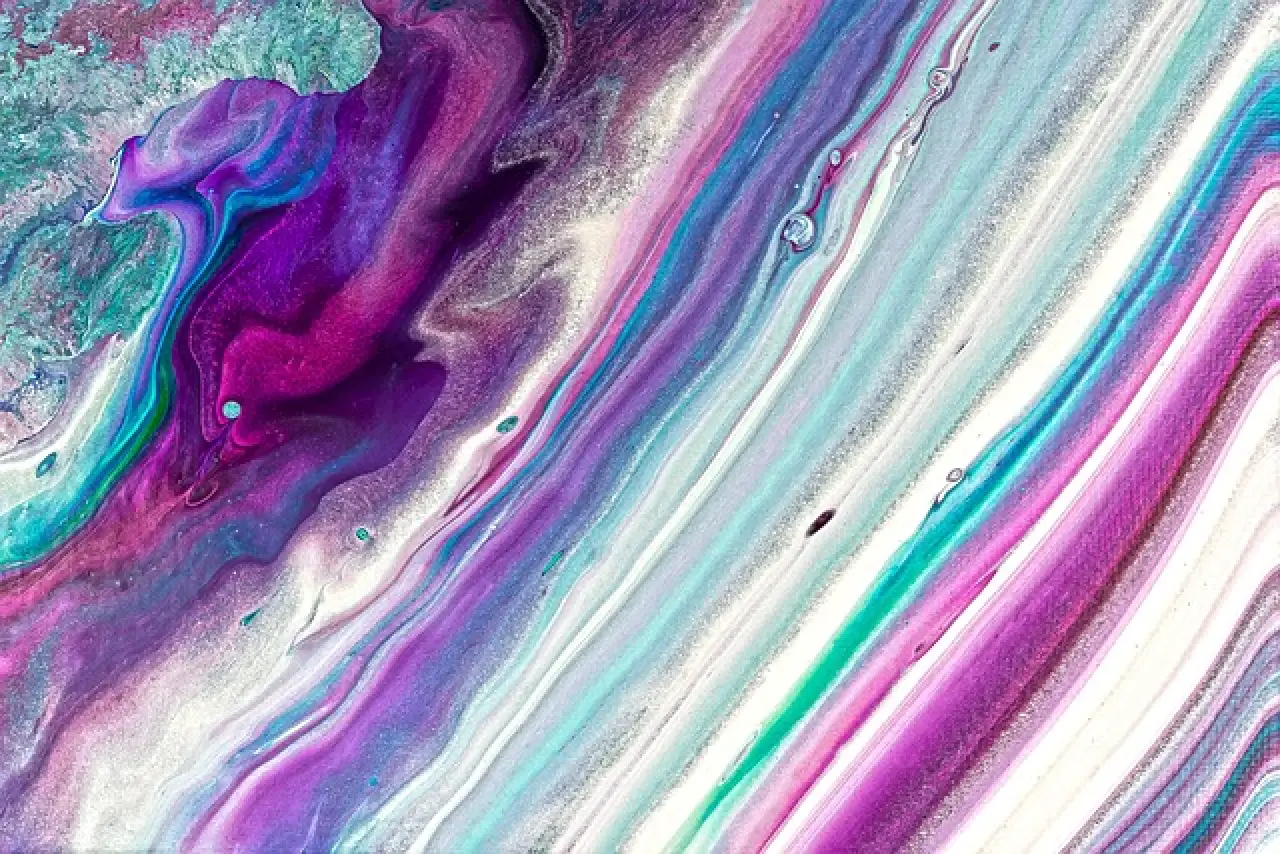
Use a Plugin to Customize the Admin Bar
There are numerous plugins available that allow you to add, remove, or reorder items in your WordPress admin area or bar. Among the most powerful plugins is Cusmin, a comprehensive tool that enables you to customize almost every aspect of your WordPress or wp admin bar area.
With Cusmin, you can delete the default WordPress logo in the toolbar, create new toolbar links, remove existing ones, and more. It offers a user-friendly interface where you can make these changes, making it an excellent option for those uncomfortable with code.
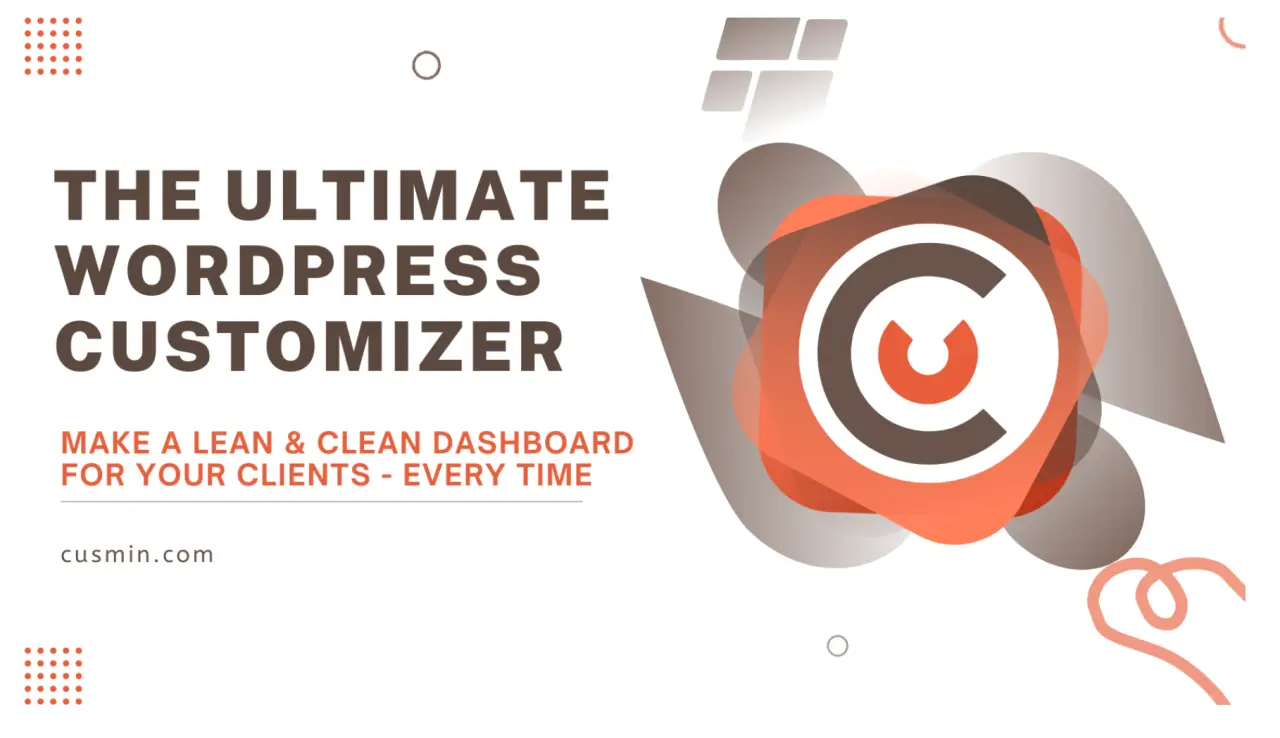
To use Cusmin to customize your admin bar, follow the steps below:
-
Install the plugin: Navigate to your WordPress dashboard, go to 'Plugins', and click 'Add New'. Click upload plugin and upload Cusmin zip file (if you don't have it already, please get one HERE)
-
Navigate to the settings: Go to admin menu > Settings > Cusmin, to open Cusmin settings
-
Navigate to admin bar tab: Open the Admin Bar tab in Cusmin to change the admin bar.
-
Customize the admin bar: Here, you can add new items, remove existing ones, and even change plugin settings. When you're satisfied with the changes, hit 'Apply' button.
Cusmin is an excellent option for those who want to customize their WordPress dashboard and admin bar without digging into code.
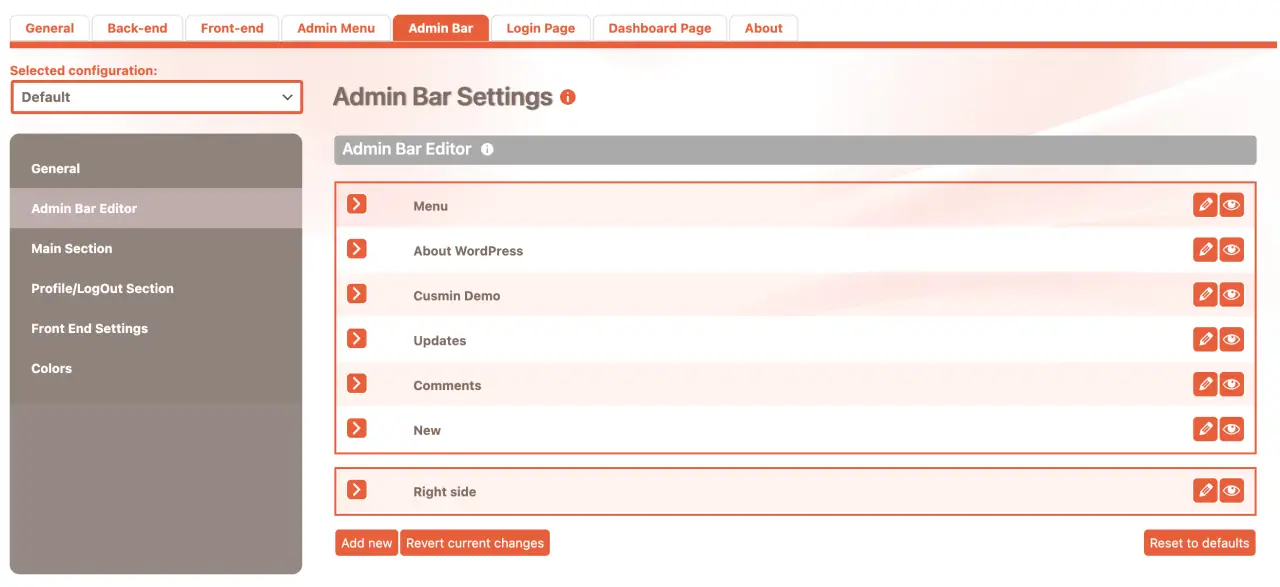
Customize the Toolbar Using Code
If you're comfortable with PHP and want more control over your WordPress admin bar, you can customize it using code. We'll be using the add_action and add_node functions to add a new item to the admin bar.
Before proceeding, ensure you have a recent backup of your website theme folder, or preferably, use a child WordPress theme to avoid losing changes when updating your WordPress' theme files. Also, you should use a preferred text editor to make these changes.
Let's take a look at the following code snippet:
<?php
add_action( 'admin_bar_menu', 'toolbar_link_to_mypage', 999 );
function toolbar_link_to_mypage( $wp_admin_bar ) {
$args = array(
'id' => 'my_page',
'title' => 'My Page',
'href' => 'http://example.com/my-page/',
'meta' => array( 'class' => 'my-toolbar-page' )
);
$wp_admin_bar->add_node( $args );
}
?>
In the above code, we define a function called toolbar_link_to_mypage, which adds a new node to the toolbar. We then use add_action to hook this function into admin_bar_menu, which is the action that WordPress uses to build the admin bar for all users. The '999' is a priority that ensures our function runs after all other functions hooked into admin_bar_menu.
Save this code in your website or active theme's functions.php file, and a new link named "My Page" will appear on your website or admin bar.
How to Remove Items from the Toolbar?
If your toolbar feels cluttered, or there are items you don't need, you can remove them. Like adding items, you can remove toolbar items using a plugin like Cusmin or through code.
Remove WordPress Admin Toolbar Items with Code
To remove items from the WordPress admin bar using code, we'll use the remove_node method of the WP_Admin_Bar class. However, to remove an item, you first need to find its ID. You can do this by inspecting the element in your web browser, which should reveal the HTML ID. Once you have this, you can use the following code snippet in your theme's functions.php file:
<?php
add_action( 'admin_bar_menu', 'remove_from_admin_bar', 999 );
function remove_from_admin_bar( $wp_admin_bar ) {
$wp_admin_bar->remove_node('my_page');
}
?>
The above code removes the "My Page" link that we added earlier. Note that if you're trying to remove a default WordPress item, you might need to look up its ID. For example, the ID for the WordPress site logo is wp-logo.
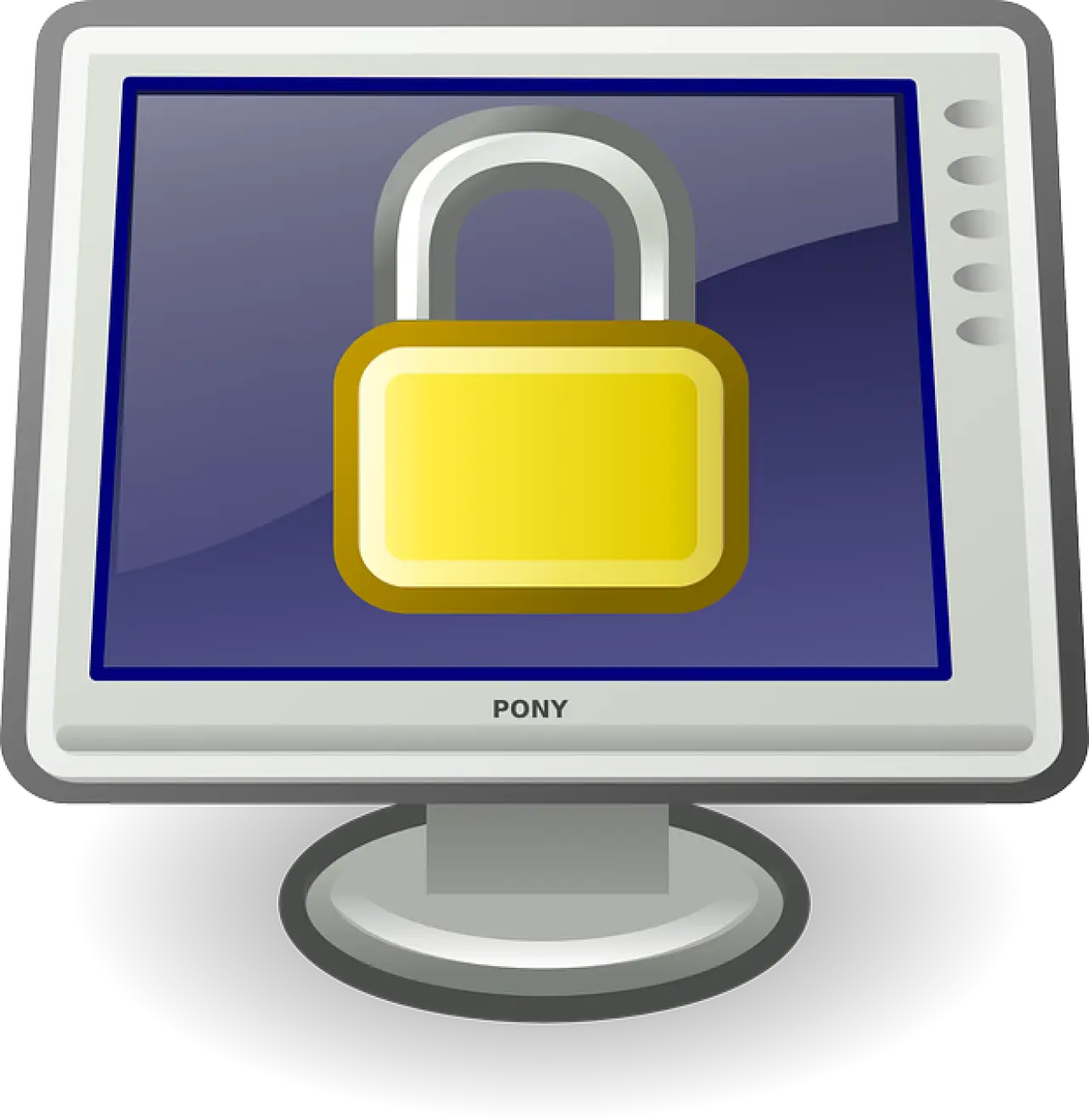
Remove Toolbar Items Using a Plugin
If you're uncomfortable with code, or if you prefer a simpler way, you can use Cusmin or a similar plugin to remove toolbar items. The process is similar to adding items. After installing and activating Cusmin, navigate to the 'Admin Bar' tab inside the plugin and select the items you want to remove.
Customizing the Appearance of the Toolbar
If you want the toolbar to better match your site's style or branding, you can customize its appearance with CSS. As with other changes, this can be done via a plugin, but for this example, we'll use code.
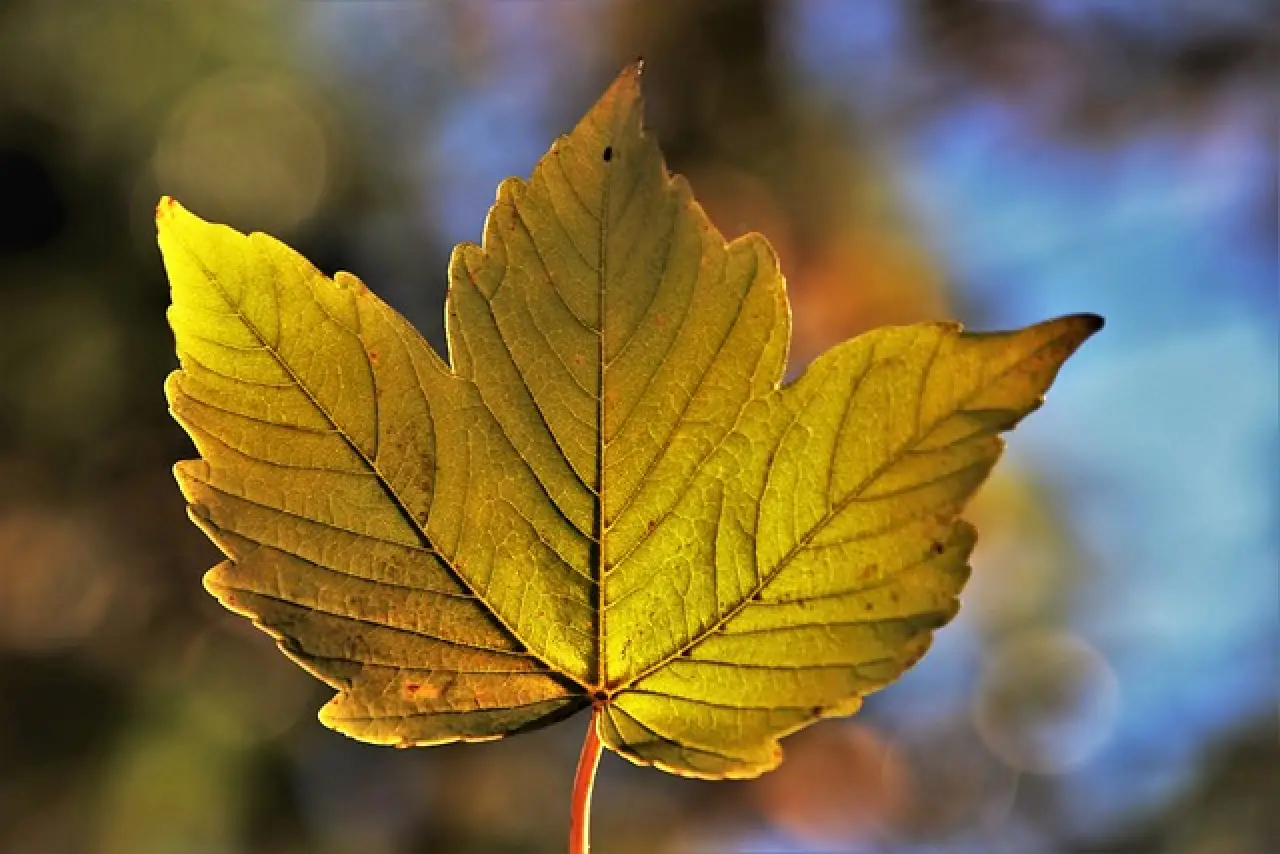
You can add the following CSS to your theme's style.css file or the "Additional CSS" section in the WordPress Customizer.
#wpadminbar {
background-color: #000;
}
The above code changes the background color of the admin bar to black (#000). You can replace "#000" with any color code to match your design.
Toolbar Permissions: Managing Who Can See What
By default, the toolbar is visible to all logged-in users and contains different options depending on the user's role. However, you might want to customize what different users can see.
This requires more complex code, usually involving the current_user_can() function to check the user role's capabilities. Alternatively, you can use a role management plugin like Members by Justin Tadlock, which lets you control what specific users in different roles can do, including what they can see in the toolbar.
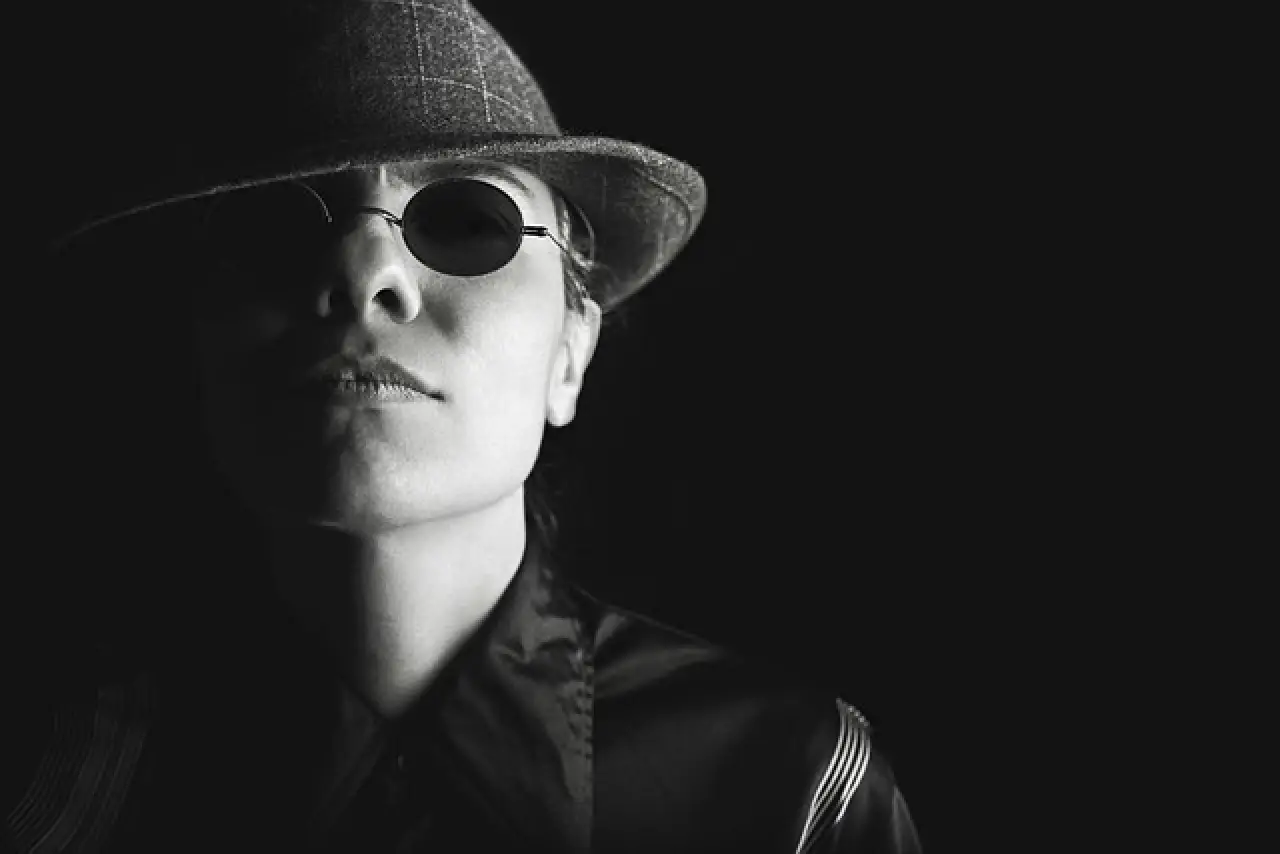
Using the Toolbar in Development
The toolbar can also be helpful when developing or debugging your site. It includes the "Debug Bar" and "Query Monitor" plugins, which add useful information to the toolbar about database queries, PHP errors, HTTP requests, and more. This is advanced usage, but it can be incredibly helpful if you're troubleshooting performance issues or bugs.
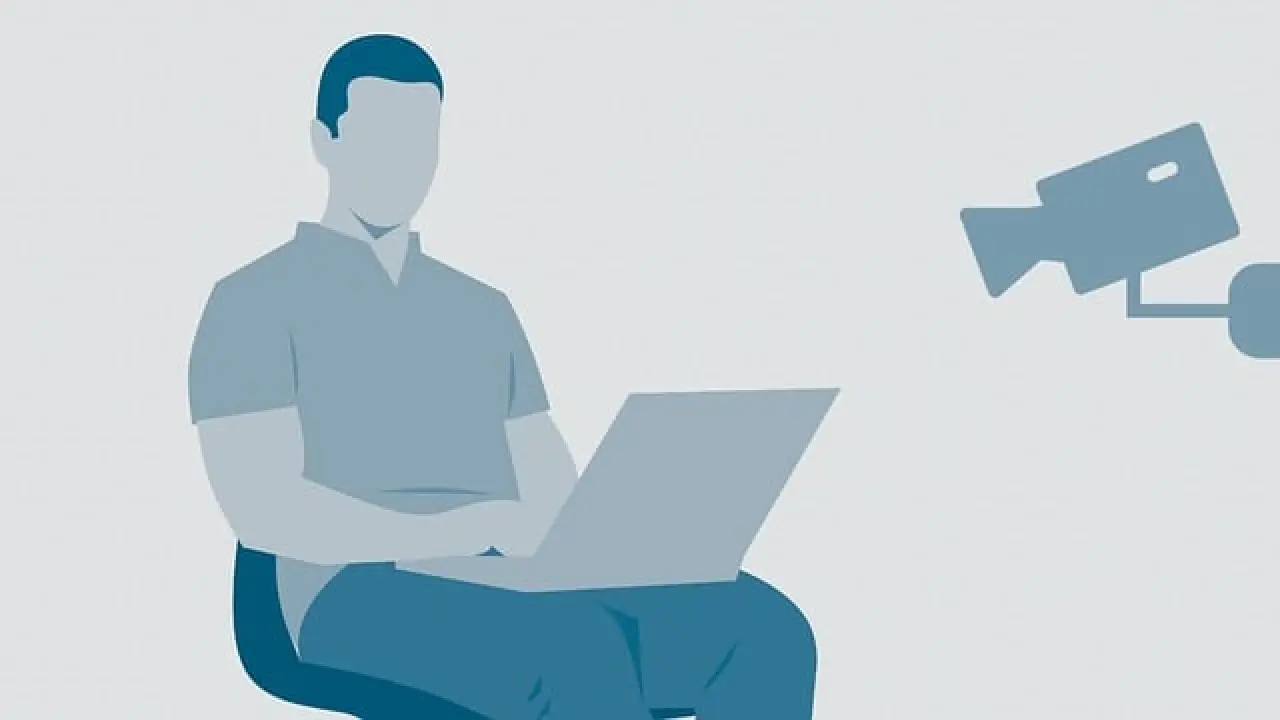
Disabling the Toolbar
Sometimes, you might want to disable the toolbar completely. Perhaps you don't find it useful, or it's covering part of your theme's design. To disable it, navigate to Users -> Your Profile in the WordPress dashboard and uncheck "Show Toolbar when viewing site". If you want to disable it for all users, you can add the following code to your current theme functions.php file:
<?php
add_filter('show_admin_bar', '__return_false');
?>
This code uses the show_admin_bar filter to return false, which hides the toolbar bar for all users only.
How to Hide Admin Bar for Mobile Users
The admin bar can be cumbersome on smaller screens such as those on mobile devices. To improve user experience for mobile visitors, you may want to hide the admin bar.
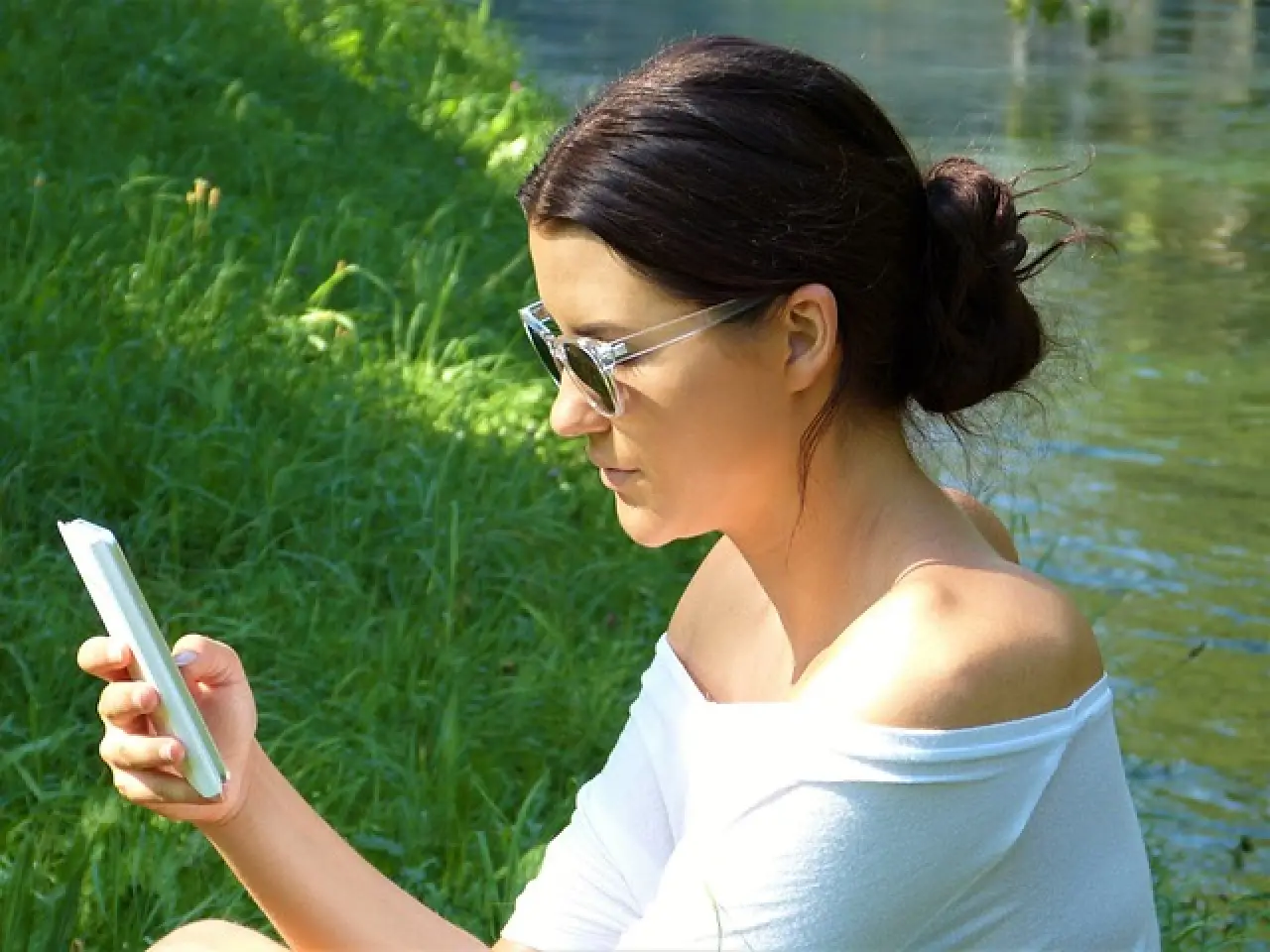
To hide the admin bar for mobile users, you can add the following code to your theme's style.css saved file or the Additional CSS section in the WordPress Customizer:
@media screen and (max-width: 782px) {
#wpadminbar { display: none; }
}
This code uses a media query to target screens with a maximum width of 782 pixels, which is roughly the breakpoint for mobile devices.
How to Add Custom Logo to the Admin Bar
To strengthen your brand identity, you may want to replace the default WordPress logo in the admin bar with your own. This can be done by utilizing the 'admin_bar_menu' action and the 'add_node' method.
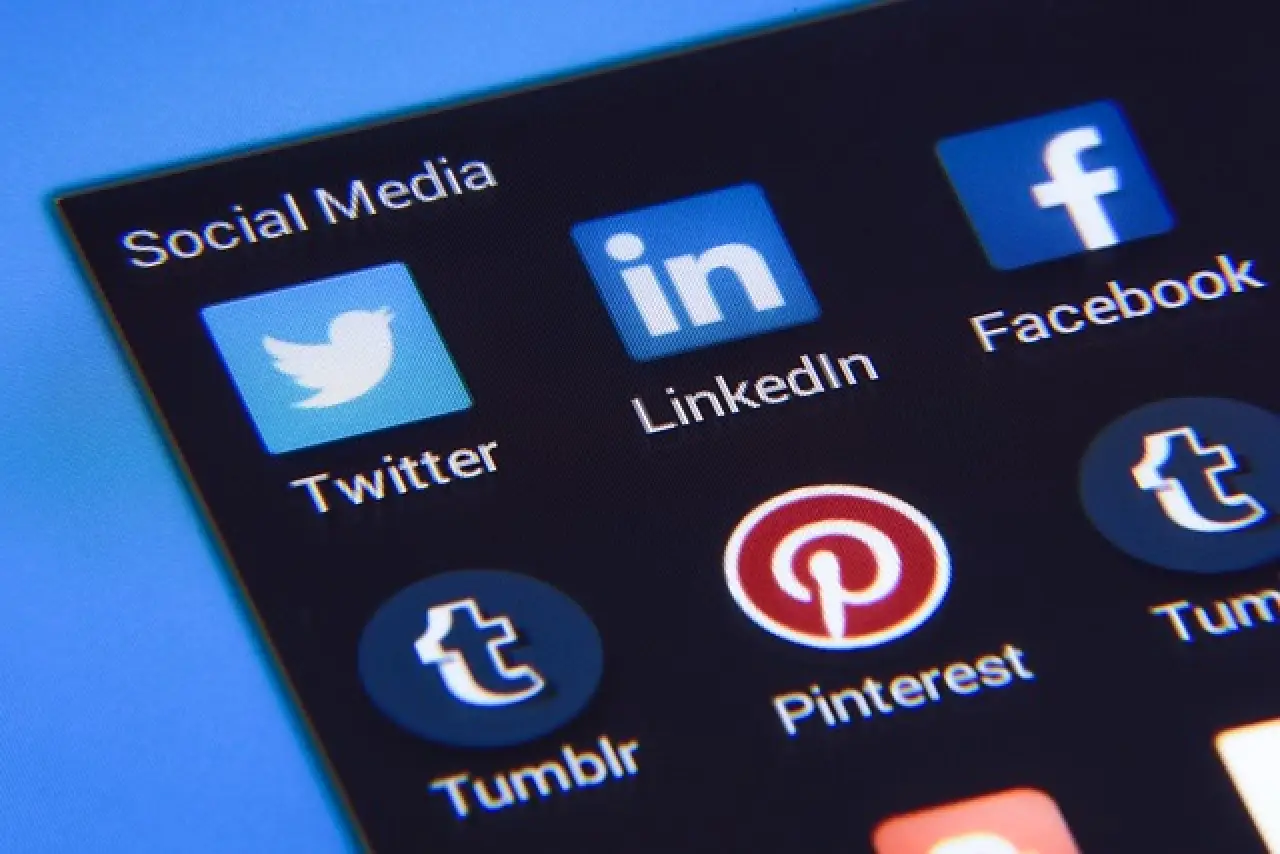
Add the following code to your theme's functions.php file:
<?php
function my_custom_logo_in_admin_bar() {
global $wp_admin_bar;
$wp_admin_bar->add_menu( array(
'id' => 'my-logo',
'title' => '<img src="' . get_bloginfo('stylesheet_directory') . '/images/custom-logo.png" alt="' . get_bloginfo('name') . '" height="28" width="28" />',
'href' => __(site_url()),
'meta' => array(
'title' => get_bloginfo('name'),
),
));
}
add_action('admin_bar_menu', 'my_custom_logo_in_admin_bar', 0);
?>
Remember to replace '/images/custom-logo.png' with the path to your logo image file within your website's theme folder.
Troubleshooting Common Issues
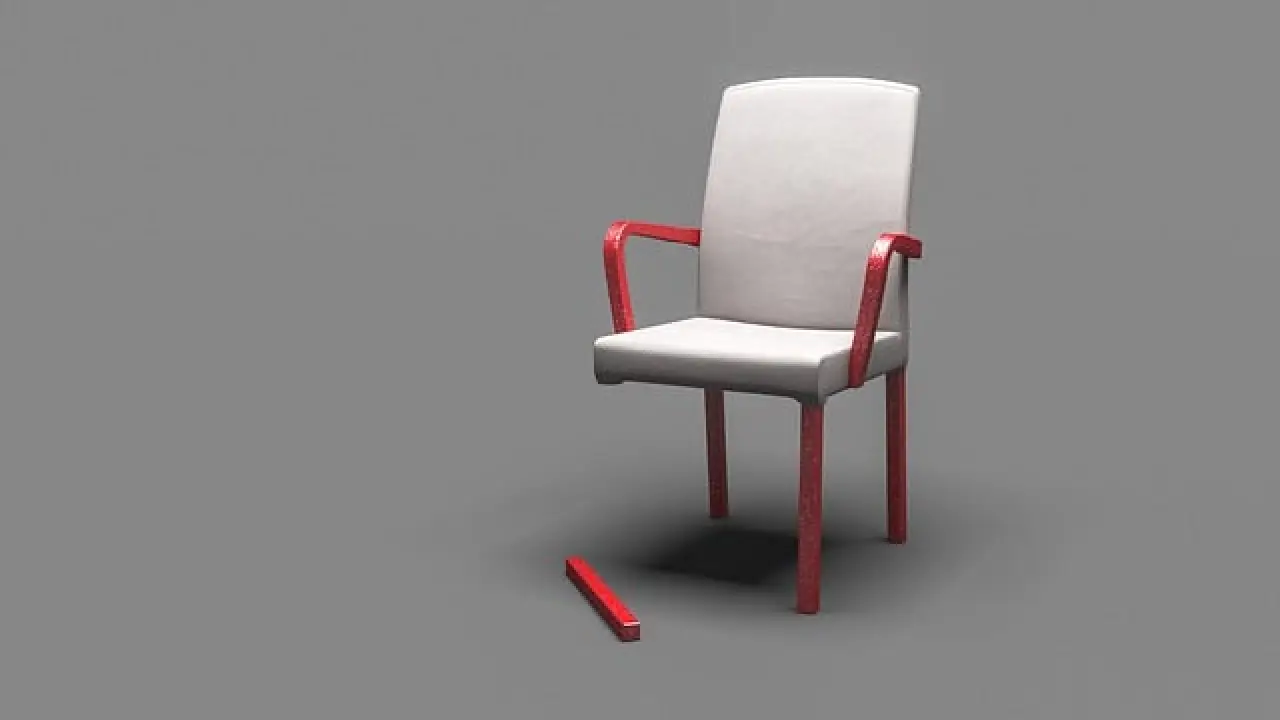
Missing Admin Bar Issue
One common issue that users face is a missing admin bar. This issue can occur due to various reasons, such as conflicts with poorly coded themes, plugin conflicts, or changes in settings.
Verify User Profile Settings
The first step to resolve the missing admin bar issue is to check your user profile settings. Navigate to 'Users' -> 'Your Profile' from your WordPress dashboard, and ensure the 'Show Toolbar when viewing site' option is checked.
Check Theme and Plugin Conflicts
If the issue persists, you might be dealing with a theme or plugin conflict. Deactivate all the plugins, and switch to the default theme. If the admin bar appears, reactivate the plugins one by one, and switch back to your original theme to identify the problematic component. Once identified, you can decide whether to keep the problematic theme or plugin or replace it with a better-coded alternative.
Admin Bar Not Displaying Properly
Sometimes, the admin bar may not display properly due to poorly coded themes or CSS conflicts. For example, if your theme has a CSS rule for a generic ID or class that the admin bar also uses, it may affect the display of the toolbar.
To troubleshoot, switch to a default theme and see if the problem persists. If switching themes solves the issue, you may need to debug your current theme's CSS to find and fix the conflict.
Managing Admin Bar Visibility
While the admin bar can be an efficient tool, not all users may need it. For instance individual users, subscribers or contributors to your site might not require access to the functionalities provided by the toolbar.
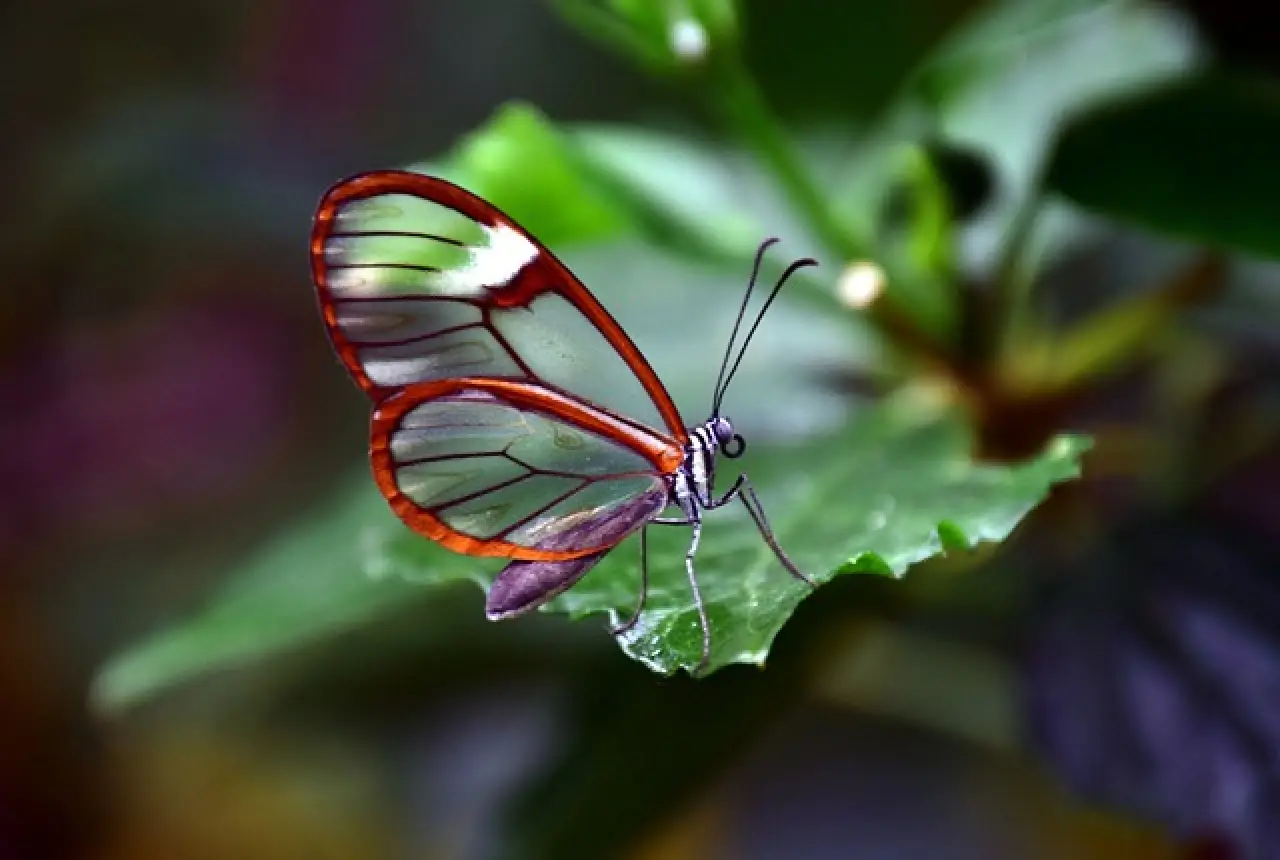
To manage visibility of user profiles based on user roles, use the following code snippet in your theme's functions.php file:
<?php
function remove_admin_bar_based_on_role() {
if (!current_user_can('administrator')) {
add_filter('show_admin_bar', '__return_false');
}
}
add_action('after_setup_theme', 'remove_admin_bar_based_on_role');
?>
This code will remove the admin bar for all users except administrators.
WordPress Dashboard Not Showing Up
Another common issue users face is the WordPress dashboard not displaying properly, or CSS not loading correctly, which might result in a white bar instead of the usual admin bar. This issue can often be resolved by adding the following code snippet to your wp-config.php file:
<?php
define('CONCATENATE_SCRIPTS', false);
?>
Before making any changes, ensure you have a backup of your website's theme folder, and make these changes using your preferred text editor.
The Admin Bar Appears on the Front End
This issue can be caused by a number of factors, including theme and plugin conflicts or errors in the WordPress core files. To troubleshoot this, deactivate all your plugins. If the admin bar disappears, reactivate the plugins one by one until the admin bar reappears, thus identifying the problematic plugin.
If plugins aren't causing the issue, switch to a default theme such as Twenty Twenty-One. If this resolves the problem, then your theme may be poorly coded or has conflicts with the admin bar.
Some Menu Items Are Missing from the Admin Bar
Missing menu items can be a sign of a plugin conflict. Deactivate all your plugins, then reactivate them one by one to pinpoint the problematic plugin. If the menu items are still missing, switch to a default theme to see if the issue is with your theme's functions only.
Another possibility could be due to user roles. Different user roles have different capabilities, hence they see different menu items in the admin bar. Make sure that the missing menu items are not user role name-specific.
The Admin Bar is Not Fixed at the Top
If your admin bar is not fixed at the top of the page as it should be, this can be due to CSS conflicts. Check your theme's CSS file for any rules that could be affecting the position of the admin bar.
A common issue is the body CSS rule. WordPress automatically adds a margin-top to the body class when the admin bar is displayed. If your theme's CSS overrides this, it could result in the admin bar not being fixed at the top.
White Bar Appears When Admin Bar is Disabled
When you hide the admin bar using the 'show_admin_bar' filter, it might leave a white bar at the top of your site. This is due to the margin added by WordPress to make room for the admin bar.
When the admin bar is removed, the margin remains and can be seen as a white bar at the top of your website. You can remove missing admin bar issue with this by adding the following CSS to your theme:
body.admin-bar #wphead {
padding-top: 0;
}
body.admin-bar #footer {
padding-top: 0;
}
JavaScript Errors Causing Admin Bar to Not Display Properly
Some admin bar features, such as the dropdown menus, rely on JavaScript to function. If there's a JavaScript error on your site, it might cause menus in the admin bar to not display properly.
You can check for JavaScript errors by using the developer tools in your browser. If you find any, the error messages will generally provide clues about the cause, which could be a plugin, theme, or WordPress core file.
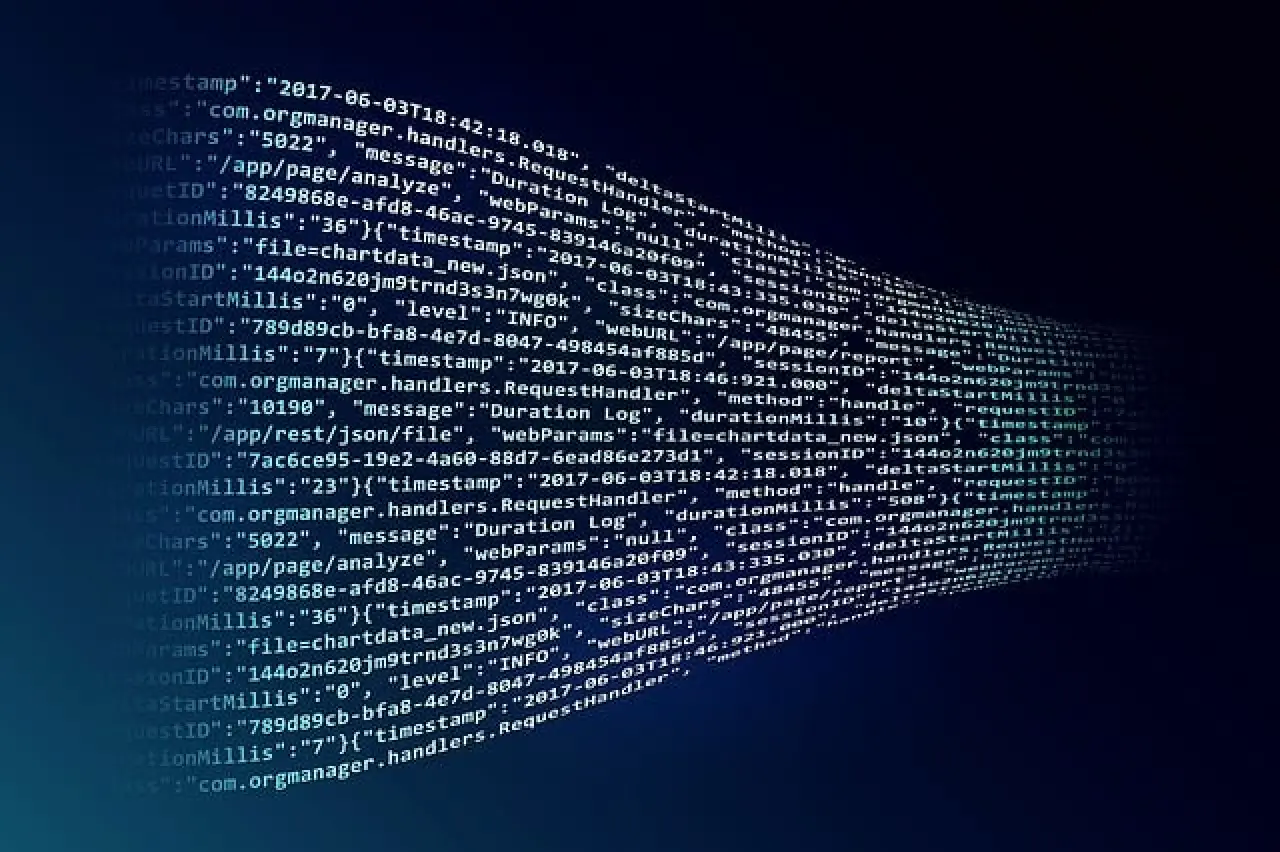
WordPress Database Errors
In some rare cases, database errors might cause issues with the admin bar. To troubleshoot this, you can enable WordPress's database repair feature. Add the following line to your wp-config.php file:
<?php
define('WP_ALLOW_REPAIR', true);
?>
Afterward, visit this URL: https://example.com/wp-admin/maint/repair.php (replace "yourwebsite.com" with your actual domain). Click the "Repair Database" button. Once the repair is done, don't forget to remove the line you added to wp-config.php.
Conclusion
The WordPress admin toolbar provides quick access to various features and settings, making your website management tasks much easier. Whether you want to add or remove items, or hide the admin bar for certain users or only users see, you can do it all with a few lines of code or a powerful plugin like Cusmin.
By customizing the WordPress admin toolbar to suit your specific needs, you not only streamline your workflows but also make your WordPress dashboard cleaner and more efficient. So, go ahead and customize your WordPress admin bar to enhance your WordPress experience.
Happy customizing!
Comments